Top 5 Java Coding Standards and Best Practices in 2025
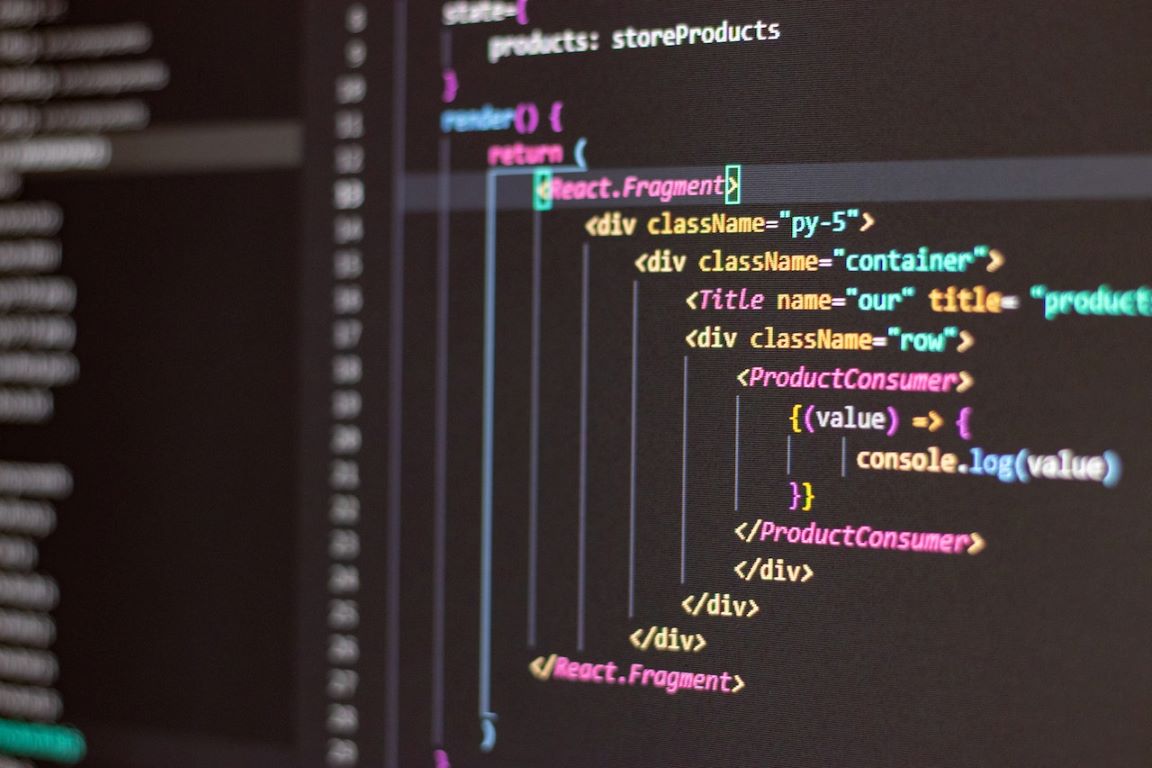
Adhering to the Java coding standards and best practices is essential as Java is an important part of the technology stack of most software development companies. The exhaustive range of features that the programming language offers, makes it an excellent choice for application development. However, with great power always comes great responsibility. In large-scale projects, developing Java applications can be complicated.
By following java coding best practices, Java development companies build high-performance and sophisticated code with ease. This blog casts light on coding standards in Java and best practices to use when implementing the language for software development.
The List of the Java Coding Best Practices
There is no single “correct” way to write Java code. However, there are certainly best practices and standards that all Java developers should follow to create quality and maintainable code. These best practices include choosing clear and descriptive variable names, using comments to document code, and writing clear and concise code. Following these rules will help make your code more readable and easier to maintain. Also, following these best practices will help ensure your code is compatible with other libraries and systems.
As a professional Java development company, we compiled a list of Java coding best practices that you should follow when coding in Java for your software development projects as well:
-
- Use Naming Standards
- Ensure that class members are in scope order
- Ensure that class members are private
- Use Underscores in Numeric Literals
- Avoid Redundant Initialization (0-false-null)
Let’s look at each of the above java coding practices in detail, below.
Using Naming Standards
It would be best if you were deliberate when handling the naming convention for your codes, and you should do this even before you write a single line of code.
As an illustration, naming conventions deal with everything related to appropriately naming a variable, constant, method, class, and interface, among other things.
When you name identifiers (methods, classes, and variables), it is essential to do so in a way that is self-explanatory, distinct, and easy to read.
Most importantly, you must always ensure to write readable codes easily understood by humans (and not just write codes to satisfy the compiler). It is a standard Java coding practice used by millions of developers.
Class Members in Scope Order
Another critical coding practice in Java you should always attempt to implement when writing is organizing member variables of a class through their scopes.
In other words, it would be ideal if you, as a Java developer, sorted each member according to the visibility of the access modifiers: private, default (package), protected, and public. A blank line must separate each group.
Class Members Should Be Private
One of the best coding standards in Java programming is to try as much as possible to reduce the accessibility of class members to the barest minimum possible.
It would be best if you use the least possible access modifier when trying to protect the class members.
Why is it highly recommended to make class members (identifiers) private? The only logical reason for practicing this is to bring out the usefulness of encapsulating information in software development.
Using Underscores in Numeric Literals
You can now write lengthy numeric literals that have an excellent readability score, many thanks to the update done in Java 7.
- Before using Underscore:
int minUploadSize = 05437326;
long debit balance = 5000000000000000L;
float pi = 3.141592653589F; - After making use of Underscore:
int minUploadSize = 05_437_326;
long debit balance = 5_000_000_000_000_000L;
float pi = 3.141_592_653_589F;
The Java declarations mentioned above demonstrate the value of utilizing underscores in numeric literals to make codes easier to read. The statement with included underscores is more readable than the other one, as shown by comparing the two statements above. It is becoming a permanent standard due to the recent Java updates.
Avoid Redundant Initialization (0-false-null)
Always refrain from initializing member variables with values like null, false, and 0 as developers. Because they are the member variables’ default initialization values in Java, you don’t necessarily need to do this.
- For example, the initialization done below is not needed; hence it is redundant:
public class Person {string name = null;
private int age = 0;
private boolean is genius = false;
}
Why Are These Java Coding Practices Considered Best Practices?
As any experienced software agency or programmer would know, these coding practices can significantly impact software quality. Well-organized and easy-to-read code is much easier to maintain and modify and can be implemented by following Java standards.
That’s the key reason not only in-house team but you can find even offshore software development company and their teams following these practices.
In addition, adhering to coding conventions can help ensure compatibility between different pieces of software. For these reasons, Java coding practices are considered best for many developers.
By following these guidelines, programmers can ensure that their code is readable, maintainable, and compatible with other software. As a result, they can save themselves a lot of time and effort in the long run.
We hope you found this article helpful! Connect with us to derive the benefit of these best practices and standards for coding Java applications in your software development projects.
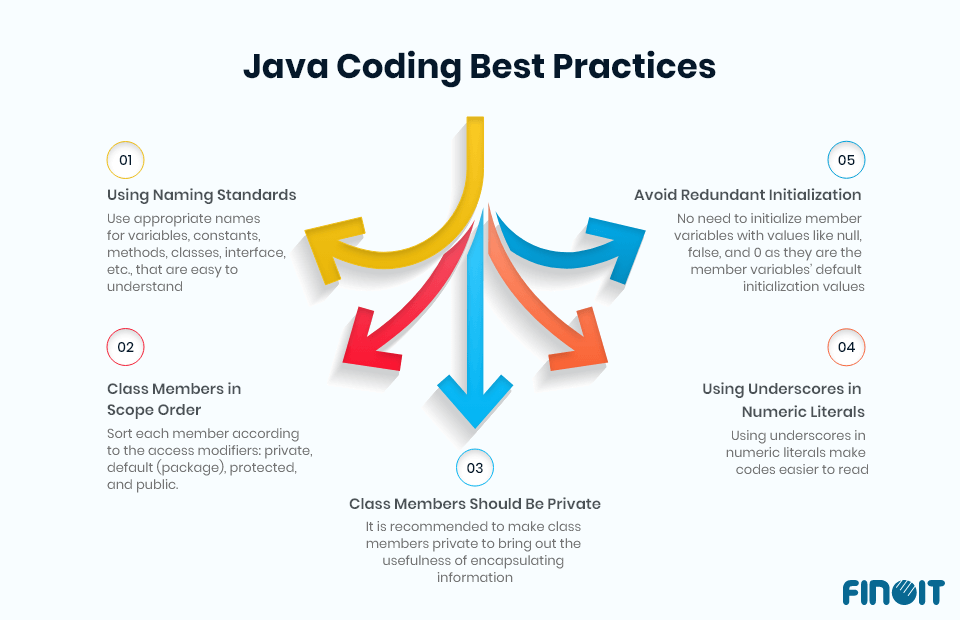
FAQ
How can I improve my Java coding standards?
There are many ways to improve your Java coding standards. Some suggestions include:
- Adhering to the principle of least privilege when it comes to access modifiers.
- Making use of underscore characters to improve the readability of lengthy numeric literals.
- Avoiding redundant initialization of member variables.
- Following established coding conventions and best practices.
Where can I practice Java coding?
There are a few ways you can practice coding in Java. One way is to use online resources on Java (MOOCS) such as Codecademy or Udemy. You can also find many free online courses that teach Java programming. Finally, another great way to learn is by working on personal projects or contributing to open source projects.
How is Java code quality measured?
The quality of Java code can be measured by its readability, maintainability, and compatibility with other software.
How vital are coding standards?
Coding standards are necessary because they help ensure the quality of your code. Adhering to coding conventions can also make your code more readable and maintainable. Furthermore, following coding standards can help ensure compatibility between different pieces of software.