9 Clean Code Java Principles For A High Performance Application
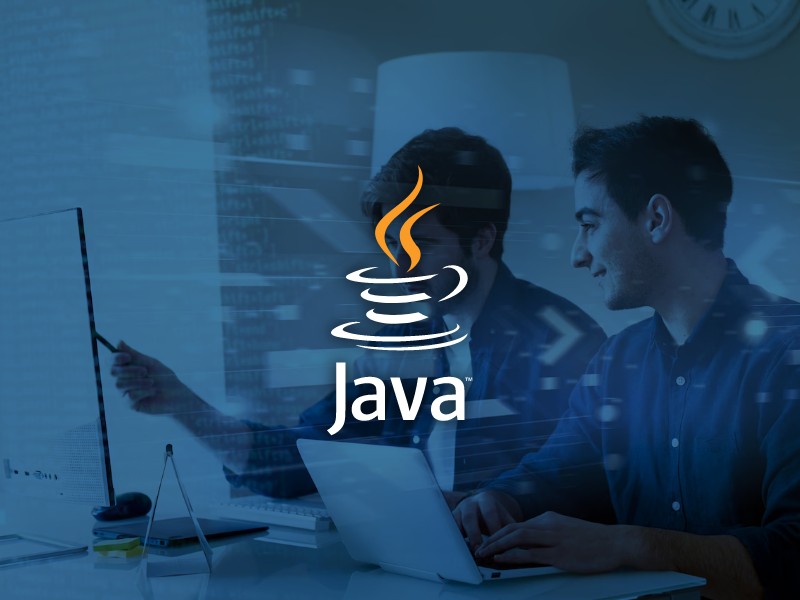
Although all computer languages share some characteristics, each has unique features and nuances. Java is a potent object-oriented language with a wealth of capabilities that make it ideal for any java development company to build business software, large-scale web applications, and mobile applications. As with any other programming language, the key to writing clean code in Java is understanding the syntax and semantics of the language, as well as following generally accepted rules and coding best practices in Java.
This blog article will look at some of the essential principles of clean code in Java. We’ll discuss what they are, why they’re crucial, and how to apply them to your code. Let’s get started!
What Is Clean Code in Java?
Clean code is straightforward to read and comprehend, as the term suggests. It is well organized and consistent and follows the standard conventions of the Java programming language.
Most importantly, clean code is free of errors and bugs. To create clean code, you need a good knowledge of the Java language and its syntax. Syntax refers to the rules that determine how any program in Java is written and then interpreted. Additionally, it would help if you had a solid grasp of object-oriented programming ideas.
But even if you are a novice programmer, you can write clean code by adhering to a few basic rules. In the long term, programming will be much simpler for you (and others) if you take the effort to write clean code.
What are the best clean coding practices in Java?
There is no single answer to the best Java practice question because different developers have different coding preferences. However, there are some standard clean coding practices that most Java developers follow:
- One common practice is to make classes and methods short and focused. It also makes the code more readable and maintainable.
- Furthermore, it is regarded as best practice to comment on the code clearly and straightforwardly. It aids in the comprehension of the code’s functionality by other developers.
Your Java code will become clearer and easier to read if you adhere to these standards.
How to Write Clean Code in Java?
In addition to the above options, some general principles can help you write clean code in Java.
Structure your code correctly
The first step to writing clean code is to structure it properly. It means dividing related code into groups and separating code that performs different functions. For example, you might have a class for storing data and another type for processing it. You may make the code easier to read and comprehend by arranging it. You also make it easier to reuse parts of the code in other projects.
As per Maven, here is a structure that programmers should follow as per clean code principles:
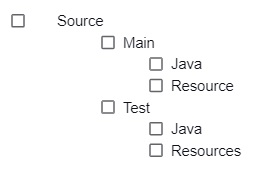
Let us understand about each directory in the structure:
Main and Test are two subfolders in the Source folders. The source files of your project will be present in the Source/Main/Java, while Source/Main/Resources will contain the resources. Similarly, the test source files and test resource files will be present in the Source/Test/Java and Source/Test/Resources folders.
Apart from Maven, you can choose a structure that you find best suited for your project. The point is that you must have a standard structure.
Use clear and descriptive names
Another primary principle is to use clear and describable names for variables, techniques, and classes. It makes it much easier for other developers to understand what your code does. It also makes it easier to find bugs. In addition, using clear and descriptive names makes it easier to change your code later.
In Java, names have to be given to classes, methods, variables, etc. The names must relate to these code components. For instance, if you need a variable that can work as an index, naming it as ‘index’ makes more sense as compared to naming it ‘a’.
When writing classes and methods, the function they perform must corroborate with the naming. For instance, if you are writing a function for forecasting, then you should name the method as Forecasting Function or on similar lines.
Define a standard Source File Structure
Different types of elements would be present in the source file structure. You should build a source file structure and follow it across the entire coding.
Here is what a well-written structure looks like:
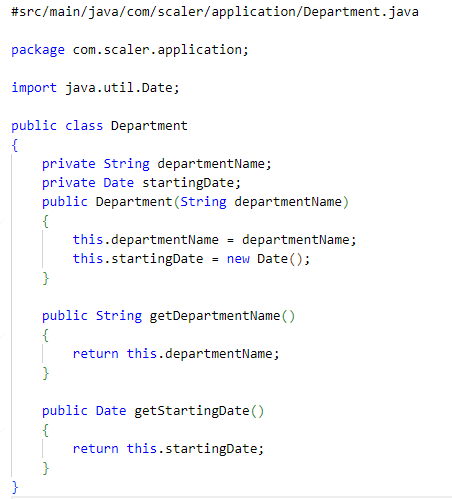
Format the code consistently
It’s also important to format your code consistently. It improves the readability of the code and makes it easier to find bugs. There are many different coding Java practices you can follow. Still, it is generally accepted that using tabs or spaces for indentation and camel cases for variable and method names is good practice.
Here is a comparison of well-followed indentation and whitespaces and when they are not taken care of:
Improper indentation and whitespaces:
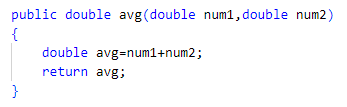
Well-followed indentation and whitespaces.
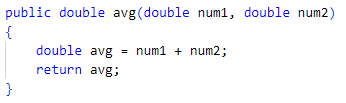
Avoid too many parameters in method
It is always a good java coding practice to optimize the number of parameters in a method. Having too many parameters in a method makes it unnecessarily complex and difficult to interpret.
Here is a difference between the two scenarios:
Too many parameters in a method:
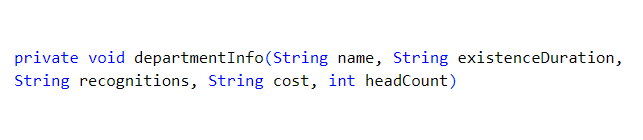
When the number of parameters is optimized:

Say no to hardcoding
Including data directly into the code can detrimentally affect the code’s performance. Termed hardcoding, this practice becomes infeasible when writing big codes. It leads to bugs and makes the code prone to errors.
Let’s say you want to determine the duration spent by an employee in a company. Instead of hardcoding the required variables around time and date, you can fetch them from classes built specifically for them. This keeps you from modifying the code for every new value.
Write well-coded logs
A well-written log is a key characteristic of a clean code written in Java. Since the programming language has permeated almost every sphere of software development, quality logs serve many purposes.
When a new programmer is onboarded in the team, with logs she can easily discover the bugs. Logs do simplify the debugging process, however, programmers must ensure to keep logs optimized, lest it decreases the processing performance.
Address duplicate code
Sometimes two or multiple methods in your Java program may have repetitive code. In such a case, the clean code Java practice is to extract the repetitive code to a separate method or class. This method or class must then be used for all instances of repetitions. This way of maintaining the code decreases the occurrence of bugs.
Do add code comments
Honed programmers add sensible comments so that when a new programmer tries to read the code, he can easily understand what function the code performs. Commenting on codes is a Java coding practice that programmers must follow strictly. When the code is uncommented, debugging becomes a time-consuming process.
Following are the two types of comments which can be used in Java programming language. These are documentation and implementation comments.
Documentation comments: These comments help in writing large programs for a software application, which helps to build a documentation API. The documentation comments are included inside /** and */.
Implementation Comments: They are used when the programmer wants to give information about the function the code is performing. There are two types of implementation comments, which are line comments and block comments.
Line comments:
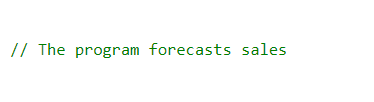
Block comments:
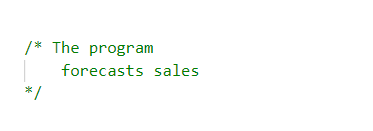
Wrapping Up
Clean code is an essential practice when writing programs. Apart from clean code being a generic concept, we saw how clean code applies in the context of Java programming. If you follow the above principles and adhere to them, you will have a high-performance code that will optimize the application development process. Along with these clean code practices, use the principles DRY (Do not repeat yourself) and KISS (Keep it simple and short) so that you avoid making your code prone to unnecessary complexities.
Coupling and Cohesion are other important dimensions of Java coding best practices. Variables, methods, classes, and other elements that are related to each other must be combined to form a single module. On the other hand, there should be less dependability amongst elements of different classes or modules. So, in Java, there should be a high cohesion but low coupling in Java code, and whether you are a software agency or individual developer, you should follow these approaches to get the high quality code.
Last but not the least, explore different tools that help to implement clean code. You can use code formatting and code analysis tools like SonarQube, SpotBugs, Veracode, Codacy, FindBugs, Coverity, PMD, etc. Get in touch with our experts to code your applications using clean code principles through such tools.
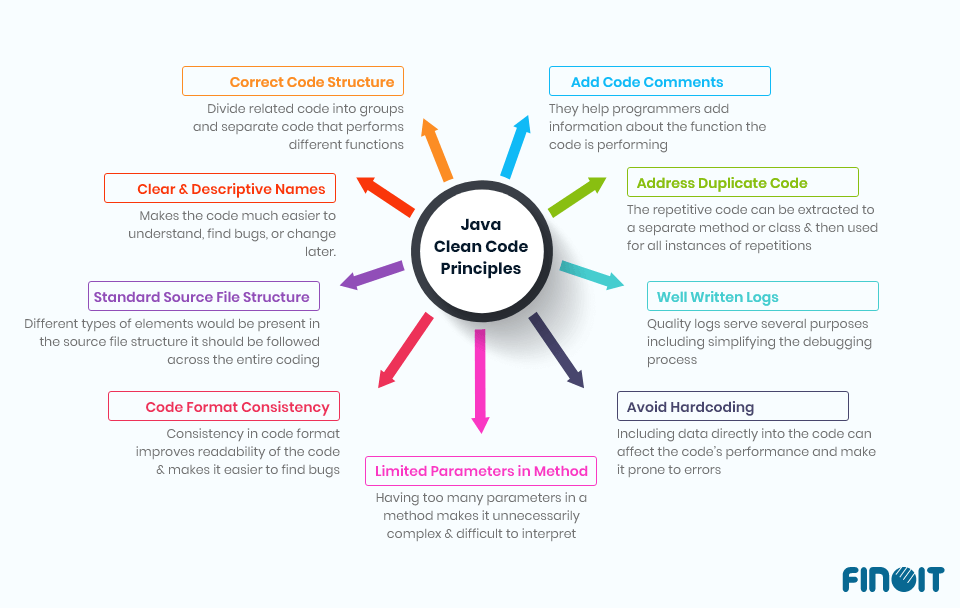
FAQs
What are your top 3 rules for writing clean code?
There is no single answer to this question, as different developers have different preferences. However, some standard clean coding practices include using clear and concise variable and method names, making classes and methods short and focused, and commenting on the code clearly and straightforwardly.
How do you write clean code?
Some general principles can help you write clean code. These include structuring your code correctly, using clear and descriptive names for variables, classes, and methods, commenting on your code clearly and concisely, and formatting the code consistently.
What are the characteristics of clean code?
Some general characteristics are often cited as being important for clean code. These include readability, maintainability, and scalability.
What is the difference between clean code and comments?
Clean code is code that is easy to read and understand. Comments are explanatory notes that are added to the code to help explain how it works.